Day Zero DRAFT
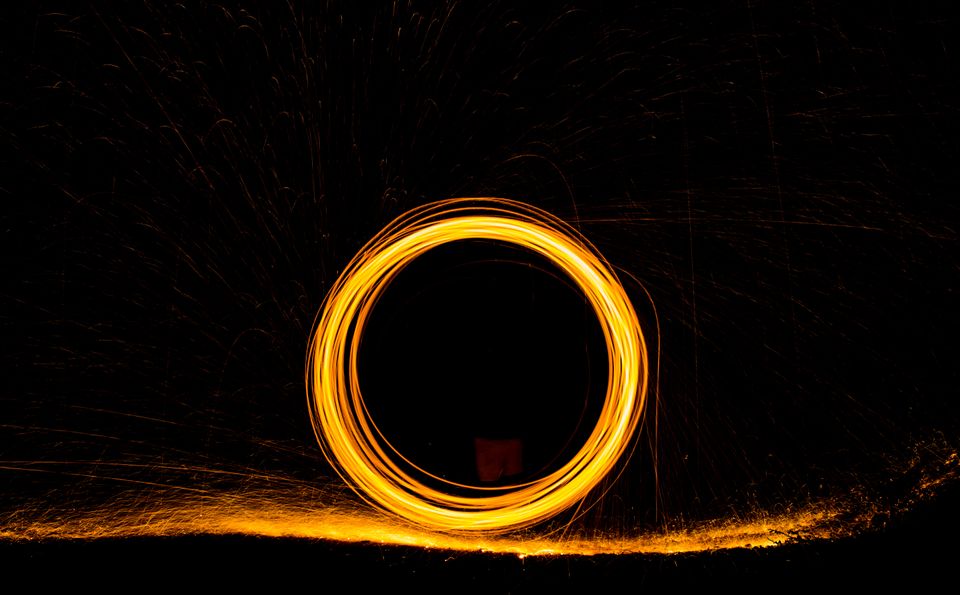
Most of us know that computers only understand 0's and 1's which is binary or base2. However, why is this? If a computer can understand 0's, 1's how come someone can write an essay or make calculations on the computer? Well, it turns out that binary can represent anything including but not limited to numbers, images, music, and text. The main reason for binary is because processors only understand electrical signals which are either on or off. However, Us humans use and rely on what is known as the Decimal number system or base10. We also use language like English or Spanish to communicate ideas. So to better illustrate how binary works lets use the base10 to represent letters in our alphabet.
A B C D E F
0 1 2 3 4 5
As you can see for each letter, a numerical value is assigned to represents that letter in the alphabet. Can you spell your name using only numbers?
3 0 11 11 0 18 -> DALLAS
Because computer processors can only understand two numbers inherently is it possible to represent base10 numbers in base2? Yes, and as said before base2 can represent anything very efficiently. In grade school, you probably used what is known as base1 to learn how to count objects. Base1 uses 1 as a representation of something like a tally. What this means is for each place value it can only represent 1x1.
In Base10 we calculate place-values by multiplying 10 by the previous place value. In unary, we multiply everything by 1 to calculate the next place value.
Group of 3 in unary -> 111
Group of 4 in unary -> 1111
Group of 1 in unary -> 1
Group of 0 in unary -> "Nothing."
Binary, on the other hand, uses both 0 and 1 to represent something. Here is an example followed by an explanation.
Group of 3 in Binary -> 11
Group of 4 in Binary -> 100
Group of 1 in Binary -> 1
Group of 0 in Binary -> 0
Binary place-values are calculated by multiplying 2 by the previous place value. However unlike our decimal system that has 10 different numbers binary only uses 2 digits to represent each place value.
1's
2's
4's
8's
16's
32's...
- Tip it's easier to read binary from right to left.
- Tip if the first digit from right to left is 0 then the number is even.
- ...8, 4, 2, 1<- 1 will always make a number odd.
- 1 0 0 1 0 1 1 0 1 -> 301
From the previous example, we illustrated Characters in the alphabet using only numbers in the base10 format. With the knowledge you have now can you create a new table using base2?
Hopefully, this helps you understand that any letter has a binary representation. Below is a code snippet in python that converts the number 13 into binary. We will go over the code in detail at a later time, but the formula for converting a number to binary is as follows.
- Divide the number by 2.
- Get the integer quotient for the next iteration.
- Get the remainder for the binary digit.
- Repeat the steps until the quotient is equal to 0.
number = 13;
binary = []
while (number > 0):
binary.insert(0, const%2)
number = number/2
print (binary)
The ASCII table was created to map a character with its binary representation. I highly suggest visiting this website for more information on the ASCII table.
Understanding the code
Code is just a set of instructions to solve a problem. The snippet of code above is known as source code, and this is human readable. Machine Code or Object code is created by compiling the source code down to something the processor understands. The great news is that when writing software one doesn't need to worry about binary. However, it's always great to know how things work on a fundamental level, so you're not left with believing a computer is a magic box.
Source code comes in many different flavors, and you've probably heard of some languages like Java, C++, Python to name a few. All languages are fundamentally the same with some being better than others for particular cases. Python is known for crunching numbers well whereas Java is excellent for creating desktop GUI applications.
Pseudocode is a made up language that only humans understand which makes it great for initial design of an algorithm without actually writing computer source code. , however, is very similar to a real language making it easy to implement in the language of your choice at a later time. We will be using this to understand the basics of coding before moving to a real langauge.
Every program you write will at the least have 3 basic qualities.
Operators
Operators tell the computer to perform mathematical or logical manipulations. Programming languages offer many different types of operators such as Arithmetic, Relational, or Logical. Most programs you write will probably need to calculate the total at checkout or check to see if someone is late on a bill.
Operator types and uses
Arithmetic
Arithmetic operators include all the standard math functions like addition, subtraction, multiplication, and division. Aside from those another Arithmetic operator modulus is used to find the remainder of the division. Although not always the case most programming languages natively can create a decimal number from a whole number, so the remainder gets dropped. For instance 5/2
would return 2 when it's 2 with a remainder of 1. If you recall the python code, we used modulus to convert a decimal number to binary.
- Addition ->
3+5
// Adds two or more numbers - Subtraction ->
5-2
// Subtracts two or more numbers - Multiplication ->
5*5
// Multiplys one or more numbers - Division ->
4/2
// Divides one or more numbers - Modulus ->
5%2
// Divides one or more numbers and returns the remainder
Relational
Relational operators are used for comparing numbers, objects, words, etc. Think about Greater than, less than, or equal to concepts as they are the same. Relational operators return true or false.
- Greater than ->
5 > 6
// Returns false - Less than ->
5 < 6
// Returns true - Greater than or equal ->
5 >= 6
// Returns false - Lessthan or equal ->
5 <= 6
// Returns true - Equal ->
7 == 7
// Returns true - Not equal ->
5 != 6
// Returns true because 5 and 6 are NOT EQUAL
Assignment && Increment
Assignment Operators allows you to assign something a value. We use a single "=" sign to illustrate this. So, for instance, I can say Birthday = 'July 31st'
and so now Birthday will always be equal to July 31st.
Increment Operators allow you to increment or decrement the value by one. For instance, let's say I was counting the number of cars that pass my house I could use the symbol ++ to increase the count by one. Likewise, if I was counting down the days till my birthday I could use -- to decrease the number by 1.
- Assign ->
Birthday = 'July 31'
// Gives Birthday the value of July 31 - Increment ->
Age++
// Age = 21; Age now equals 22 - Decrement ->
Days_Till_Birthday--
// Days_Till_Birthday = 10; Days_Till_Birthday now equals 9
Logical
Logical operators are used in determining if something is true or false. They take boolean (true or false, 0 or 1, yes or no) values as input and return boolean values as output. For instance, suppose I sleep in if I'm on vacation or it's the weekend. Otherwise, i wake up early. In this case, you could say If on Vacation OR it's the weekend let me sleep
-
AND returns TRUE if and only if both sides are true
-
OR returns TRUE if one or both sides are true
-
NOT Returns TRUE if something is false for instance I can take a break if NOT in_a_meeting
-
AND ->
Vacation && Weekend
// && represents AND but some langauges use the actual word AND -
OR ->
Vacation || Weekend
// || represents AND but some langauges use the actual word OR -
NOT ->
!Vacation
// ! represents NOT but some langauges use the actual word NOT
Conditional Statements
Conditions allow the computer to make decisions. Conditions always return True or False. For example in a computer game If lives == 0 then "Game Over"
Conditional statements make use of operators to make this decision. In programming there will be manytimes when one action is prefered over another. Remember the logical operator example? You may have an alarm application that automatically sets an alarm based on what is true. Another scenerio could be writing a program to grade a paper and assign a letter grade. You certianly wouldnt just want a pass for fail.
if (grade >= 90) return 'A';
else if (grade < 90 && grade >= 80) return 'B';
else if (grade < 80 && grade >= 70) return 'C';
else return 'D';
when programming a video game you may want to adjust the computer difficulty based on a players skill level.
if (player_death > 20) difficulty = 'easy';
if (player_death < 5 && level > 10) difficulty = 'hard';
If you are programing a ATM machine you may want to chose to dispense money if the customer has the funds in his or her account.
if (withdraw_ammount <= available_funds) dispense(withdraw_ammount);
else say('Sorry Insufficient funds.');
The idea is you will need to make choices and the cpu is reliant on the programmer to write condtions on how to make those decision. Take note the computer doesn't care if it's the correct decision or not so you must test your conditions thoroughly. In my programming career most bugs come from conditional statements. So a suggestion is to talk it out and make use of a whiteboard or pen & paper to illustrate your thought process before writing code. You wouldn't want the ATM to dispense money if the customer didn't have the funds avaliable so it can help to write a test case first to assert the logic.
assertFalse(Withdraw_ammount > Available_funds);
assertTrue(Withdraw_ammount <= Available_funds);
Loops
Currently writing
// Psuedocode example
// Real world example