Hashing
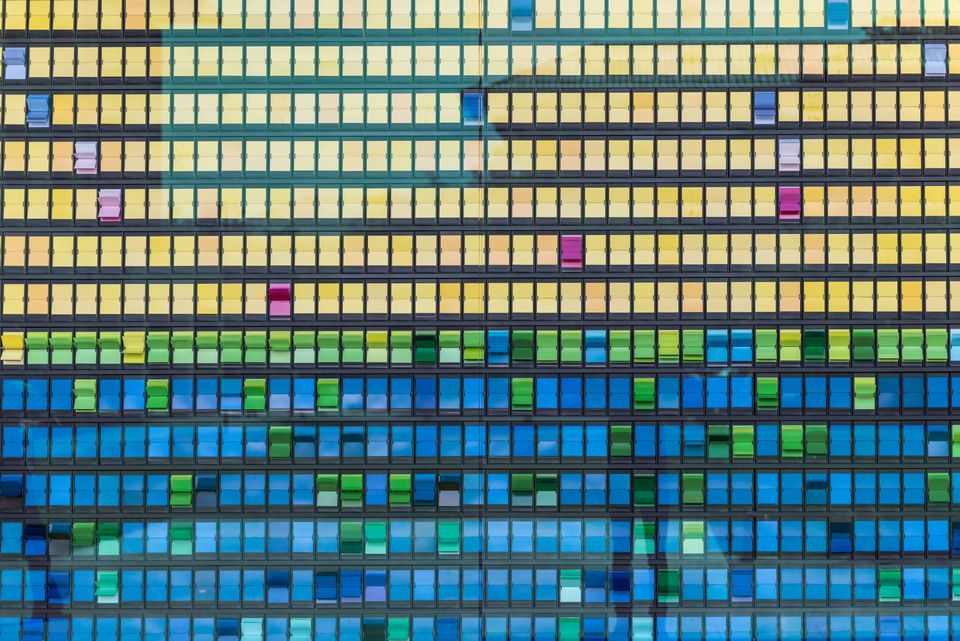
Before we get into hashing lets talk about arrays, Arrays are very effecient data structure that doesnt need to iterate to find the location of a value long as you know the index. So lets say you have a list of 10 usernames and your array consist of 1000 users. Worst case scenerio you iterate 1000 times and then perform a calculation, insert, delete... list goes on. Could there possibly be a better way to still have direct indexing?
Hashing saves the day
Code
function createHashTable(max) {
return Array(...Array.apply(max)).map(() => {});
}
function createHash(key) {
let hash = 0;
for (let i = 0; i < key.length; i = i + 1){
hash += key.charCodeAt(i);
}
return hash;
}
const myArr = createHashTable(10000);
myArr[createHash('Hello world')] = 'Hello world';