The Staircase problem
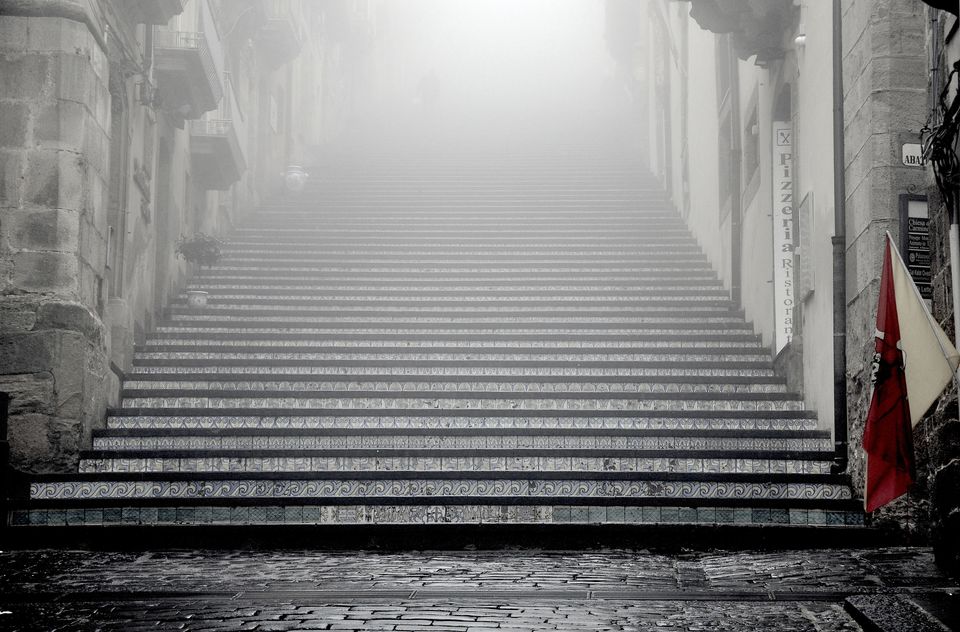
The following problem is from hacker rank...
Consider a staircase of size : n
Observe that its base and height are both equal to , and the image is drawn using # symbols and spaces. The last line is not preceded by any spaces.
Write a program that prints a staircase of size .
Input Format
A single integer, , denoting the size of the staircase.
Output Format
Print a staircase of size using # symbols and spaces.
Note: The last line must have spaces in it.
Sample Input
6
Sample Output
#
##
###
####
#####
######
Explanation
The staircase is right-aligned, composed of # symbols and spaces, and has a height and width of .
My solution using recursion
function createString(str, ns, n) {
if (ns > 0) return createString(str+" ", ns-1, n-1);
else if (n > 0 && ns === 0) return createString(str+"#", ns, n-1);
else if (n === 0 && ns === 0) return str;
}
// Complete the staircase function below.
function staircase(n) {
let ns = n-1;
while (ns >= 0) {
console.log(createString("", ns, n));
ns--;
}
}